らくらくマウスのようなボタンを押すことでポインタを動かす装置を作成します。
仕様は4方向移動、左ボタン、右ボタン、ポインタ速度5段階というものです。らくらくマウスとの違いはスクロールボタン(ホイール)が無い、4方向しか移動できない、クリックのロックができない等、機能は限定的です。
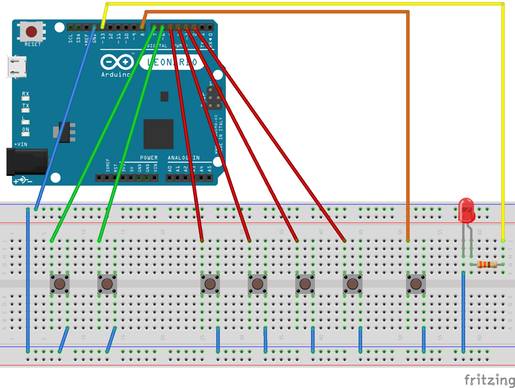
左から「左クリック」「右クリック」「左」「下」「上」「右」「速度調整」スイッチです。
Arduino Leonardo の2番ピンを右移動スイッチ、3番ピンを上移動スイッチ、4番ピンを下移動スイッチ、5番ピンを左移動スイッチと接続します。
6番ピンを右クリック用スイッチ、7番ピンを左クリック用スイッチ、8番ピンを速度調整用スイッチ、GNDと各スイッチの反対側の接点をつなぎます。
速度確認用のLEDはなくても構いません。取り付ける場合は13番ピンと接続します。LEDには電気の流れる向きがあります。またLEDに合った抵抗が必要になりますのでご注意ください。
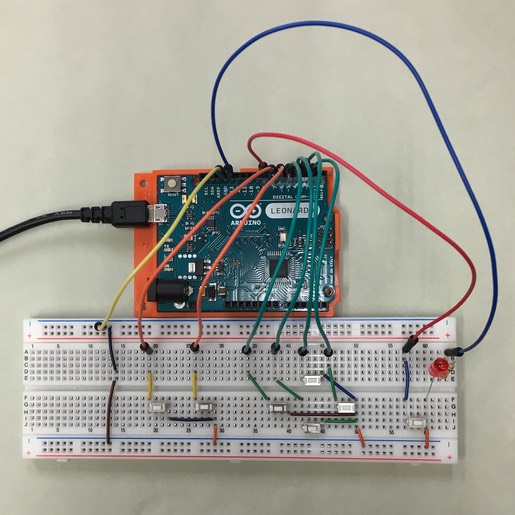
ブレッドボードで再現しました。移動用の4つのスイッチは十字に配置してあります。右側のLEDはすぐ近くのスイッチ(1番右にあるスイッチ)を押すと1〜5回点滅しポインターの速度が変わります。
以下のコードを転送します。
/* 開発環境のサンプルスケッチ「ButtonMouseControl」を参考にしました */ #include "Mouse.h" // 各ボタンの定義 const int rightButton = 2; const int upButton = 3; const int downButton = 4; const int leftButton = 5; const int leftClick = 7; const int rightClick = 6; const int speedButton = 8; const int led =13; int range = 5; // output range of X or Y movement; affects movement speed int responseDelay = 10; // response delay of the mouse, in ms void setup() { // initialize the buttons' inputs: pinMode(upButton, INPUT_PULLUP); pinMode(downButton, INPUT_PULLUP); pinMode(leftButton, INPUT_PULLUP); pinMode(rightButton, INPUT_PULLUP); pinMode(leftClick, INPUT_PULLUP); pinMode(rightClick, INPUT_PULLUP); pinMode(speedButton, INPUT_PULLUP); pinMode(led, OUTPUT); // initialize mouse control: Mouse.begin(); // Serial.begin(9600); } void loop() { // read the buttons: int upState = digitalRead(upButton); int downState = digitalRead(downButton); int rightState = digitalRead(rightButton); int leftState = digitalRead(leftButton); int LclickState = digitalRead(leftClick); int RclickState = digitalRead(rightClick); int speedState = digitalRead(speedButton); if (speedState == LOW){ range--; if (range == 0){ range = 5; } for (int i = range; i >= 1; i--){ digitalWrite(led, HIGH); delay(300); digitalWrite(led,LOW); delay(100); } } // calculate the movement distance based on the button states: int xDistance = (leftState - rightState) * range; int yDistance = (upState - downState) * range; // if X or Y is non-zero, move: if ((xDistance != 0) || (yDistance != 0)) { Mouse.move(xDistance, yDistance, 0); } // if the mouse button is pressed: if (LclickState == LOW) { // if the mouse is not pressed, press it: if (!Mouse.isPressed(MOUSE_LEFT)) { Mouse.press(MOUSE_LEFT); } } // else the mouse button is not pressed: else { // if the mouse is pressed, release it: if (Mouse.isPressed(MOUSE_LEFT)) { Mouse.release(MOUSE_LEFT); } } // if the mouse button is pressed: if (RclickState == LOW) { // if the mouse is not pressed, press it: if (!Mouse.isPressed(MOUSE_RIGHT)) { Mouse.press(MOUSE_RIGHT); } } // else the mouse button is not pressed: else { // if the mouse is pressed, release it: if (Mouse.isPressed(MOUSE_RIGHT)) { Mouse.release(MOUSE_RIGHT); } } // a delay so the mouse doesn't move too fast: delay(responseDelay); }
コードに2カ所ある ”range”の値を増減することでポインターの移動速度を変えることができます。